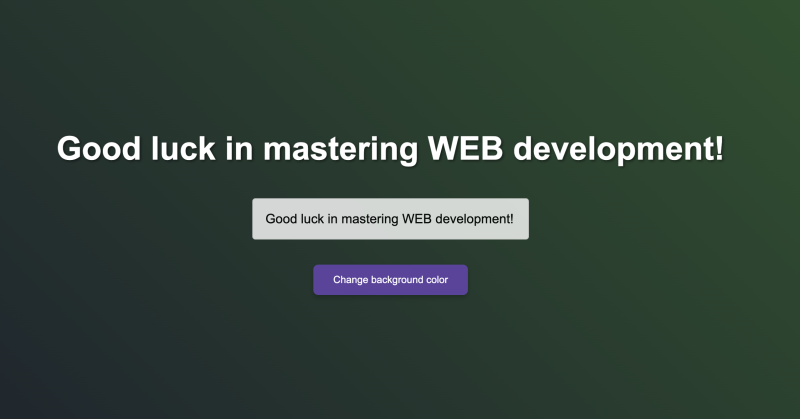
Dynamic text change on a page using JavaScript
In this lesson, we will continue exploring JavaScript and apply our knowledge in practice to create dynamic user interaction. We will add an input field to the page, and every time the text in this field changes, our heading will be updated in real-time. This will not only give you more experience working with the Document Object Model (DOM), but also demonstrate how easily interactive elements can be created using JavaScript.
Introduction:
In this lesson, we will learn how to change text on the page in real time using JavaScript. We will be working with form elements and the DOM (Document Object Model). This is a simple yet powerful exercise that allows for easy creation of dynamic web pages and, most importantly, interacting with the user.
Step 1: Let's update our HTML page
First, let's add a text field (<input>) between the heading and the button. This field will be used for entering text that will change the content of the heading.
Here is how our HTML will look:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello World Page</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1 class="animated-title" id="dynamicText">Hello World!</h1>
<input type="text" id="textInput" placeholder="Enter text here.." />
<button id="changeColorButton">Change background color</button>
<script src="script.js"></script>
</body>
</html>
Step 2: Adding JavaScript to handle changes
Now let's add the JavaScript code that will track changes in the input field and update the title text.
In our script.js file, let's add the following:
const dynamicText = document.getElementById("dynamicText");
const textInput = document.getElementById("textInput");
textInput.addEventListener("input", function() {
dynamicText.textContent = textInput.value;
});
document.getElementById("changeColorButton").addEventListener("click", function() {
document.body.style.background = `linear-gradient(45deg, #${Math.floor(Math.random()*16777215).toString(16)}, #${Math.floor(Math.random()*16777215).toString(16)})`;
});
What we have done here:
- Added an event listener to the input field, so that every time the text changes in the field, the text in the heading is updated.
- All changes happen instantly, creating a dynamic interaction effect with the user.
Step 3: Style for the new element
Let's add some styles to the input field to make it look nice on the page:
body {
background: linear-gradient(to right, #ff7e5f, #feb47b);
font-family: 'Arial', sans-serif;
color: white;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
flex-direction: column;
}
h1 {
font-size: 3rem;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.5);
animation: pulse 2s infinite;
}
input {
padding: 20px;
font-size: 1.3rem;
border-radius: 5px;
border: 1px solid #ddd;
margin: 20px 0;
width: 80%;
max-width: 400px;
outline: none;
opacity: 0.8;
}
button {
background-color: #5d439e;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 20px;
cursor: pointer;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
transition: background-color 0.3s, transform 0.3s ease;
}
button:hover {
background-color: #4a3380;
transform: translateY(-2px);
}
button:active {
background-color: #3e8e41;
transform: translateY(2px);
}
@keyframes pulse {
0%, 100% {
transform: scale(1);
}
50% {
transform: scale(1.1);
}
}
Step 4: Testing
Now that we have updated the HTML, CSS, and JavaScript, it's time to test! Open the page in a browser, and you will see how the text in the heading changes depending on what you type in the input field. If you click the button, the background of the page will change to a random gradient.
Conclusion
Congratulations! You have just created your first dynamic page that changes content in real time. This is a great example of how JavaScript can be used to interact with users, making the page interactive.
What's next?
This lesson was the last in a small Quick Start cycle. In a few simple steps, you have already familiarized yourself with the basics of working with the DOM, events, and user interactions. We started with basic concepts and created several interactive elements.
But this is just the beginning! In the next lesson, we will begin developing a real project where we will learn new technologies, tools, and capabilities. Get ready to create a full-fledged web application with real logic and functionality!