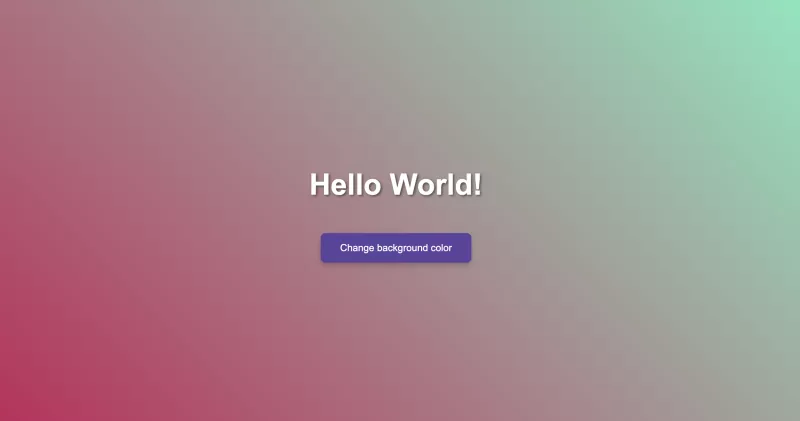
Hello World comes to life: Introduction to JavaScript
In the previous lessons, we created a simple page with the phrase "Hello World!" and enhanced it using CSS. Now it's time to add some interactivity using JavaScript! In this lesson, we will learn how to change the background of the page using a button. Let's go through step by step how to do this.
1. HTML: Page Structure
Let's start by creating the structure of the page, which will include a header and a button for changing the background. Here is how our HTML code will look:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello World Page</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1 class="animated-title">Hello World!</h1>
<button id="changeColorButton">Change background color</button>
<script src="script.js"></script>
</body>
</html>
Here:
- We have added a button with the ID changeColorButton, which will change the background of the page.
- The h1 heading contains the phrase "Hello World!" and has a class for animation.
2. CSS: Page Styling
Now let's add styles to the page to make it look appealing. In this section, we will create a gradient background, style the heading and button, as well as add animation to the text and a hover effect on the button.
body {
background: linear-gradient(to right, #ff7e5f, #feb47b);
font-family: 'Arial', sans-serif;
color: white;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
flex-direction: column;
}
h1 {
font-size: 3rem;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.5);
animation: pulse 2s infinite;
}
button {
background-color: #5d439e;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 20px;
cursor: pointer;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
transition: background-color 0.3s, transform 0.3s ease;
}
button:hover {
background-color: #4a3380;
transform: translateY(-2px);
}
button:active {
background-color: #3e8e41;
transform: translateY(2px);
}
@keyframes pulse {
0%, 100% {
transform: scale(1);
}
50% {
transform: scale(1.1);
}
}
Here:
- Gradient Background: We used a linear gradient to create a smooth transition between colors from pink to orange.
- Header Animation: Added a "pulsation" animation to make the header look dynamic and attract attention.
- Button: Made the button attractive with hover and click effects.
3. JavaScript: Adding Interactivity
Now it's time to add JavaScript. We will use a button to change the page's background. Each time a user clicks the button, the background will change colors to a random gradient.
document.getElementById("changeColorButton").addEventListener("click", function() {
document.body.style.background = `linear-gradient(45deg, #${Math.floor(Math.random()*16777215).toString(16)}, #${Math.floor(Math.random()*16777215).toString(16)})`;
});
In this code:
- We attach an event handler to the button with the ID changeColorButton.
- Upon each click on the button, the background of the page will change its color to a random gradient.
Result
Now your page looks not only beautiful but also interactive. The "Hello World!" heading pulsates, and with each click on the button, the background of the page will change to a random gradient.
Conclusion
Congratulations! You have just added interactivity to your project using JavaScript. In the next lessons, we will continue working with JavaScript to create even more interesting and dynamic web pages.
Join us in the next lesson where we will learn about working with forms and how to handle user data using JavaScript.
🚀 Don't forget to practice and experiment with the code!